Managing your Postmark templates with Github using Travis CI or CircleCI
Postmark’s templating system comes with a web editor to manage your email templates, but sometimes it's more convenient to use your own code editor while also storing your templates in version control. Our CLI tool makes this all possible.
This guide walks you through how to store your Postmark templates in Github and use Travis CI or CircleCI to automatically push them to Postmark.
If you’re not familiar with continuous integration (CI) and continuous delivery (CD), no need to worry. In a nutshell, these tools let developers run automated processes against their code and deploy it somewhere. A common scenario is for developers to run automated tests each time they push their code to Github. If the tests fail for whatever reason, the CI/CD tool notifies the developer so they can fix the issue. And if the tests pass, their code gets deployed to their server.
![Flow chart illustrating basic CI/CD workflow. Code → Github → CI/CD → Did tests pass? [YES] → Deploy code [NO] → Notify developers Flow chart illustrating basic CI/CD workflow. Code → Github → CI/CD → Did tests pass? [YES] → Deploy code [NO] → Notify developers](https://craft-assets.postmarkapp.com/blog/_705x485_crop_center-center_10_none/ci-chart@2x.png)
This is a very basic example, but some workflows can get pretty complex depending on the application. Luckily, we’re here to help you get up and running with a simple workflow. Let’s jump into it!
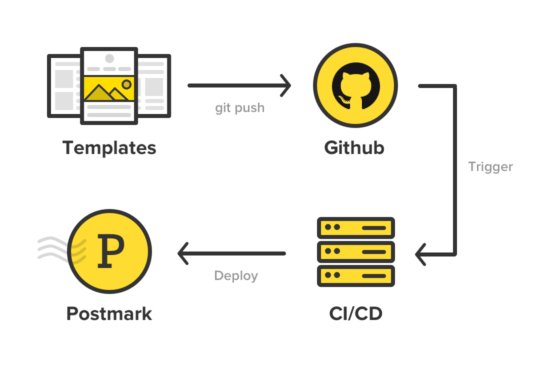
Setting up your Github repository #
First, you’ll need to set up a Github repository for your templates. Feel free to skip these steps if your repository is already set up.
- Create a Github repository. Check out Github’s help doc for instructions.
- Clone the repository to your desktop. Check out Github’s help doc for instructions.
Pulling your templates from Postmark #
Once your repository is set up, you need to pull your templates from Postmark to your local repository. But before you get started, make sure that node.js is installed.
1. Open up your terminal, and install the Postmark CLI tool:
npm i postmark-cli -g
2. Run the Postmark command to make sure it’s installed. If you see a help screen, then you’re good to go:
postmark
3. Navigate to your git repository and pull your templates:
cd ~/Projects/your-repo
postmark templates pull ./emails
The pull
command accepts the path of where you want to save your templates. In our example, we are putting the templates in ./emails
, but feel free to pass in a different path if you’re integrating into an existing project.
The CLI tool will ask you for your server token to authenticate. You can find your server token on the credentials page under your Postmark server. Once authenticated, your Postmark templates will be downloaded to your local git repository.
4. Next, let’s push your templates up to Github:
git add .
git commit -m "Initial commit of Postmark templates"
git push
Setting up CI/CD #
We’ll need to set up a CI/CD tool to push the templates from your Github repository to Postmark. Travis CI is popular option for open source projects, whereas CircleCI is more suited for private projects. Both have their own pros and cons based on OS support, number of concurrent jobs, and language support, but either tool is sufficient for what we’re doing.
Setting up Travis CI #
If you don’t already have a Travis CI account, sign up at travis-ci.org and authorize your Github account.
Once you’re logged into the dashboard, head over to the Settings page. You can find it by hovering over your avatar in the navigation:
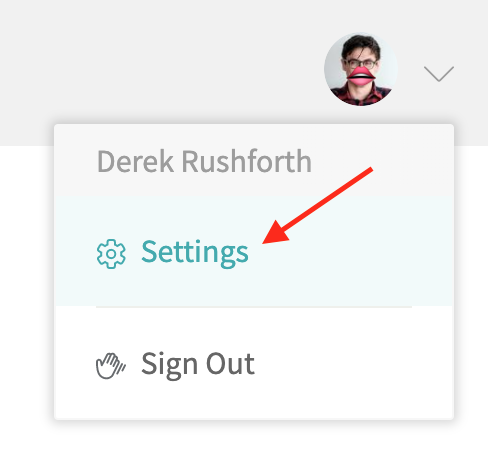
Find your Github repository and click the toggle to enable it:

Next, click on the Settings button next to the repository. Scroll down to the Environment Variables section and add a variable called POSTMARK_SERVER_TOKEN
. Enter your Postmark server token as the value.

The Postmark CLI tool automatically detects this environment variable to authenticate server-level requests. Make sure to keep Display value in build log disabled so that your server token does not get exposed in the logs.
Next, head over to your code editor and add a file called .travis.yml
to your project root and paste this config into the file:
language: node_js
node_js:
- "lts/*"
script: true
before_deploy:
- npm i postmark-cli -g
deploy:
provider: script
script: postmark templates push ./emails -f
on:
branch: master
This config provides Travis CI with instructions on how to handle the workflow. Let’s go over what it does:
language
- Our CLI tool uses node.js, so we’re making sure it’s availablenode_js
- Define’s the node.js version. The latest LTS version of node.js will work just fine.before_deploy
- Installs the Postmark CLI dependency before the deployment processdeploy
- Tells Travis CI how to handle the deploymentprovider
- Travis CI supports a long list of providers, but we just need a script for our deploymentscript
- Where the push command is executedpostmark templates push
- This command accepts the relative path of the templates. We are also passing in the force (-f
) flag. By default, this command will ask users to confirm the push, but since it's executed inside of a build container, user confirmation isn’t possible. The force flag simply disables the confirmation.branch
- Specifies that templates are only pushed to Postmark whenever a push is made to the master git branch.
Let’s commit the Travis CI config to the master branch in your github repository:
git add .
git commit -m "Add Travis CI config"
git push
Travis CI will automatically detect your push and run the deployment. Head over to the Travis CI dashboard and click on your repository:

This will take you to the logs where you can see what happened during the build. You can even use the logs to see which templates were pushed to Postmark. If everything was successful, you should see a Deploying application step containing the list of pushed templates:
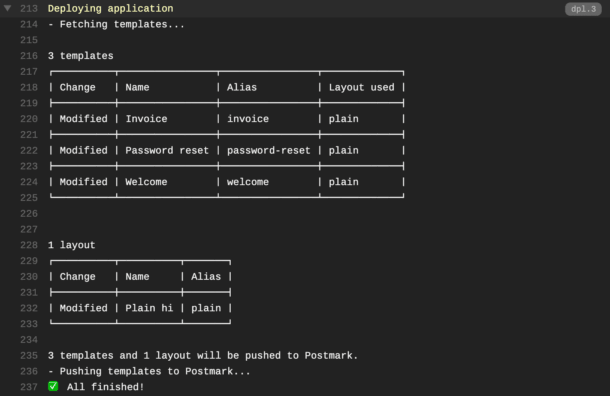
If you want to get fancy, you can even send custom email notifications each time certain steps are executed. I find it useful to send an email notification each time my templates are pushed:
after_deploy:
- postmark email raw -f=fromemail@example.com -t=youremail@example.com --subject="[Travis CI] Templates pushed" --text="Templates have been pushed to Postmark - $TRAVIS_BUILD_WEB_URL"
This utilizes the after_deploy step which is part of Travis CI’s job lifecycle. This step executes the postmark email raw
command and inserts the build URL into the --text
argument so that you can easily view the logs in Travis CI. Don’t forget to update the From email (-f
) to a valid Sender Signature or email address on a verified domain. And enter the email address of where you want to send the notifications in the -t
argument.
You’re all ready to go! I recommend checking out Travis CI’s help docs for more info on how to customize your workflow.
Setting up CircleCI #
If you don’t already have a CircleCI account, sign up at circleci.com and authorize your Github account.
From your code editor, create a folder named .circleci
in your project root. Then add a file named config.yml
to that folder. Paste this starter config into the file contents:
version: 2
jobs:
build:
branches:
only: master
machine: true
steps:
- checkout
- run:
name: Install Postmark-cli
command: |
npm i postmark-cli -g
- run:
name: Push templates to Postmark
command: |
postmark templates push ./emails -f
This config provides CircleCI with instructions on how to handle the workflow. Let’s go over what it does:
version
- Defines which config version to usejobs
- Lets you define specific tasks for a workflow. In our case, we’re only running thebuild
job to push our templates. You can also execute multiple jobs as part of a workflow if your process is more complex.branches
- Only run the build process on the master branchmachine
- Specify the type of environment to run the workflow on. We’ll use the default machine provided by CircleCI.steps
- List of steps to be performed in this jobcheckout
- Helper step provided by CircleCI used to checkout the source code so that the templates can be accessedrun
- Step type that lets you name and execute scripts. In our case, we start off by installing the Postmark CLI as a global dependency using npm, and then follow up by pushing the templates to Postmark.postmark templates push
- This command accepts the relative path of the templates. We are also passing in the force (-f
) flag. By default, this command will ask users to confirm the push, but since it's executed inside of a build container, user confirmation isn’t possible. The force flag simply disables the confirmation.
Next, let’s commit and push the CircleCI config to the master branch:
git add .
git commit -m "Add CircleCI config"
git push
Head back to CircleCI’s web app and click on the Add projects link in the navigation. Look for your repository and click on the Set Up Project button:

This will take you to a set up page with a bunch of boilerplate config options. Under the Language section, select Node:

Scroll down to the instructions section and click on the Start building button:

This will trigger a build, but it will fail since we haven’t added an environment variable for your server token yet.
Head over to the Settings page, then select the Projects from the sub navigation. Find your project and click on the little cog icon:

Click on Environment variables from the sub navigation and add a variable named POSTMARK_SERVER_TOKEN
. Enter your Postmark server token as the value:
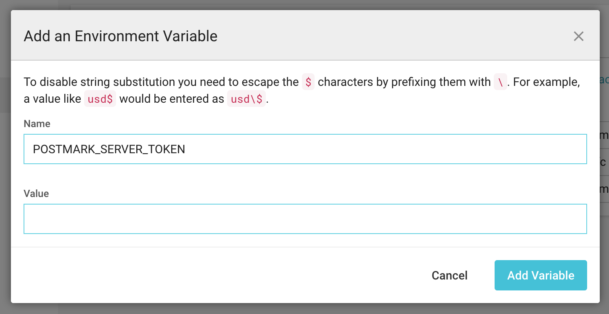
The Postmark CLI tool automatically detects this environment variable to authenticate server-level requests.
Next, we will trigger a build to make sure everything works. Click on the Jobs link in the navigation and you will see the build that failed. Click on the title of your repository:

This will take you to the job details page. Click on the Rebuild button in the top right:

Once the build is finished, head back to the jobs page and click on your repository title:

From here you will be able to see all the details about the build. If you’re curious about which templates were pushed, scroll down to the test summary and click on the Push templates to Postmark button:
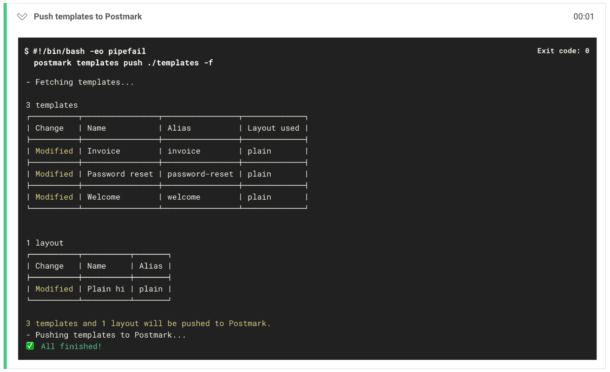
As with Travis CI, you can even send custom email notifications each time certain steps are executed. I find it useful to send an email notification after my templates are pushed. Add this step after the push command:
- run:
name: Send notification email
command: |
postmark email raw -f=fromemail@example.com -t=youremail@example.com --subject="[CircleCI] Templates pushed" --text="Templates have been pushed to Postmark - $CIRCLE_BUILD_URL"
This step executes the postmark email raw
command and inserts the build URL into the --text
argument so that you can easily view the logs in CircleCI. Don’t forget to update the From email (-f
) to use a valid Sender Signature or email address on a verified domain. And enter the email address of where you want to send the notifications in the -t
argument.
You’re all ready to go! I recommend checking out CircleCI’s help docs for more info on how to customize your workflow.
Using a bash script #
Using a CI/CD tool sounds great and all, but what if you want to just push your templates locally using a bash script? This is another feasible option, but it’s tough to manage for larger teams.
1. Add a bash file (.sh
) to your project and name it whatever you want:
#!/bin/bash
export POSTMARK_SERVER_TOKEN=Enter your token
# Push templates from current directory
postmark templates push ./emails
# Send notification email (Optional)
postmark email raw -f=fromemail@example.com -t=youremail@example.com --subject="Templates pushed" --text="Templates have been pushed to Postmark"
2. Enter your Postmark server token on line 2. The Postmark CLI tool automatically detects this environment variable to authenticate server-level requests. We recommend that you also add this script to .gitignore
since it contains your server token.
3. Run your script:
sh yourscript.sh
4. By default, you will be asked to confirm your changes to prevent any accidental pushes:
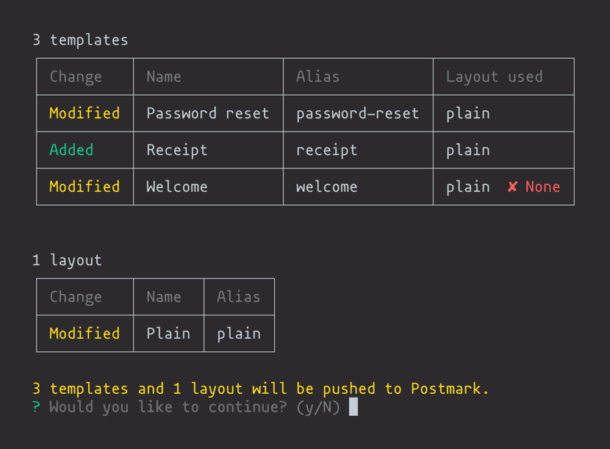
That’s it! You can even use this script with deployment platforms like DeployBot or Buddy.
A note of caution #
Once your templates are stored in Github, we recommend that you avoid making changes in our in-app web editor or you run the risk of template versions getting out of sync. If this happens by accident, follow these instructions:
1. Open your terminal and navigate to your project’s template directory:
cd ~/Projects/your-repo
2. Make sure that you have no changes in your current working tree:
git status
3. You should see something like this:
nothing to commit, working tree clean
If you see that there are changed files, you need to stash your files and proceed.
4. Remove your templates from the project:
rm -r ./emails
If a template was removed in Postmark, but not in your Github repository, the templates pull
command will not remove it. So we need to make sure you are working from a clean slate since the pull
command is only additive.
5. Pull your templates to the project:
postmark templates pull ./emails
6. Enter your Postmark server token to authenticate the request.
7. Review your changes:
git status
8. If everything looks good, push to your git repository:
git add .
git commit -m "Sync templates from Postmark"
git push
Your Github repository is now up to date with the latest template versions in Postmark.
What’s next? #
We hope you find this helpful. This guide should serve as a starting point to setting up a template development workflow, but if you’re looking to use more advanced tooling, we recommend MailMason. With MailMason, you can use Grunt tasks, Handlebars, and SASS to streamline your template development workflow.
P.S. We highly recommend that you make use of Postmark Layouts. It's a way to define common elements like CSS, Headers, and Footers, and reuse those Layouts across many Templates. It will help save you a bunch of time time when developing templates.