How to use Laravel Mailbox with Postmark
Some weeks ago, a new Laravel package library for processing inbound email messages called Laravel Mailbox was published. Having worked on projects with this type of functionality previously, I was intrigued by how it allowed different transactional SaaS services to be used with a single API.
Laravel Mailbox shipped supporting three drivers: Mailgun, Sendgrid, and Local (the last one for development purposes). I came across this new package on my Twitter timeline and asked its creator, Marcel Pociot, about the possibility of adding extra drivers, and in my particular case, supporting Postmark’s API. He explained that it was possible and that it would make a great addition to the package.
Since his reply, I began looking for a way to contribute to the project, and by extension, to the Laravel community as a whole. The Laravel community is excellent. Everyone is caring, open to dialogue, and helpful. I felt this was an opportunity to give back to the amazing people I’ve come to respect and appreciate.
I started browsing the code base of the package and began adding the new feature that would allow using Postmark’s service for processing inbound email messages in Laravel. It was a challenging experience since I didn’t have too much knowledge of package development for the Laravel framework. With the help of some online tutorials and Marcel’s guidance, I was able to have the feature ready for submission. Days later, Marcel announced it was part of the package library and had first-hand support.
I couldn’t believe I had pulled this one off. I was so happy my effort was worthy of other fellow developers and could be used to facilitate their work in the future. It felt great and satisfying to be able to give back to the community, and I cannot stress the value, experience, and knowledge gained while contributing to the project.
Let's walk through how to use Laravel Mailbox with Postmark.
Configure Postmark for inbound mail processing #
The Laravel Mailbox official website includes detailed information on how to install the library in your Laravel project. In this section, I would like to extend on the installation notes with how to use the library with the new Postmark driver.
After installing Laravel Mailbox in your project, we can start configuring Postmark’s settings for inbound mail processing.
First, we need to set up some environment variables in our project to instruct Laravel Mailbox to use Postmark’s driver. These variables are:
MAILBOX_DRIVER
, which should have a value of “postmark”MAILBOX_HTTP_USERNAME
, which can be any username you’d like. The default for Laravel Mailbox is “laravel-mailbox” so I’ve decided to keep it.MAILBOX_HTTP_PASSWORD
, which should be a random password used to identify the incoming message authority (more on this later).
To generate the MAILBOX_HTTP_PASSWORD
variable, we will use a simple command utility available in Linux and Mac OS distributions. Open up a terminal window and type the following command:
openssl rand -hex 32
This command will generate a random string that we can use for our MAILBOX_HTTP_PASSWORD
variable.

Your environment variables should look something like this:
MAILBOX_DRIVER=postmark
MAILBOX_HTTP_USERNAME=laravel-mailbox
MAILBOX_HTTP_PASSWORD=7bf8815b30f2980c4f597828cc785a8cf4a4027a9dc14444b9aac7409220ee4b
Next, we will log into our Postmark account to configure sending inbound email messages to our server and start processing them. If you don’t have one account, you can register one for free.
In your Postmark account, go into one of your existing servers or create a new one in the Servers section. In this case, I’ll be using “My First Server” which is the default server Postmark creates for you.
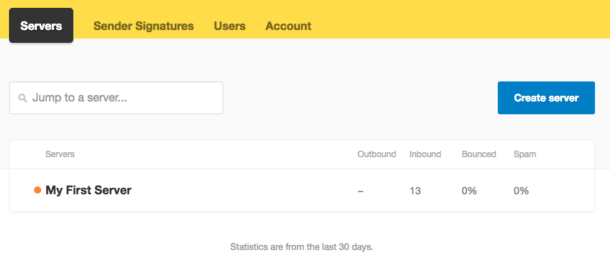
Inside the Postmark server details, head on to the Settings tab for Inbound messages and look for the Inbound webhook field.
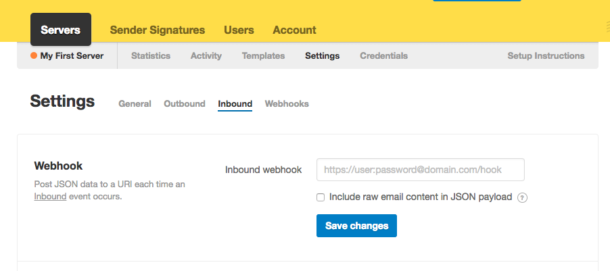
In this field, we must configure a Laravel web route from our project where we want our messages to be delivered once they hit Postmark’s servers. The inbound webhook should be a live web route (accessible through the internet) since Postmark will check the URL once it’s configured.
The web route we are going to configure should have the following structure:
https://YOUR-USERNAME:YOUR-PASSWORD@YOUR-DOMAIN/laravel-mailbox/postmark
Where:
YOUR-USERNAME
should be the same username defined in our environment variable,MAILBOX_HTTP_USERNAME
. In this case, “laravel-mailbox”.YOUR-PASSWORD
should be the same password used in theMAILBOX_HTTP_PASSWORD
environment variable.YOUR-DOMAIN
should reflect your application's domain. For example, “myawesomeapp.com”.
The last segments of the URL, laravel-mailbox/postmark
, is where Laravel Mailbox is going to receive messages when using the Postmark driver.
NOTE: I will be using ngrok.io to configure my local development machine as a live server in order to continue the tutorial. You can also use this type of configuration during development. We will be using 70b680b7.ngrok.io
as our app domain.
Once we identify the bits and pieces, our inbound webhook URL should look like this:
https://laravel-mailbox:7bf8815b30f2980c4f597828cc785a8cf4a4027a9dc14444b9aac7409220ee4b@70b680b7.ngrok.io/laravel-mailbox/postmark
Use this URL in the inbound webhook field. You must also check the “Include raw email content in JSON payload” option since it is required by Laravel Mailbox in order to retrieve and process the original MIME message. Press the Check button to continue. You’ll notice a successful message with the configuration we have made.
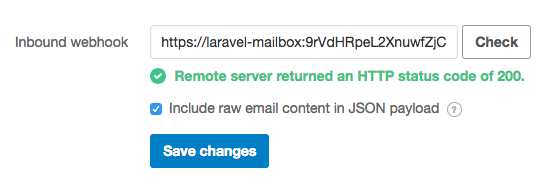
Next, save the changes.
Continuing in our Postmark account, let’s go into the Credentials section. From here, copy the Inbound email address provided by Postmark. This email address will allow us to test our inbound email processing using Laravel Mailbox.
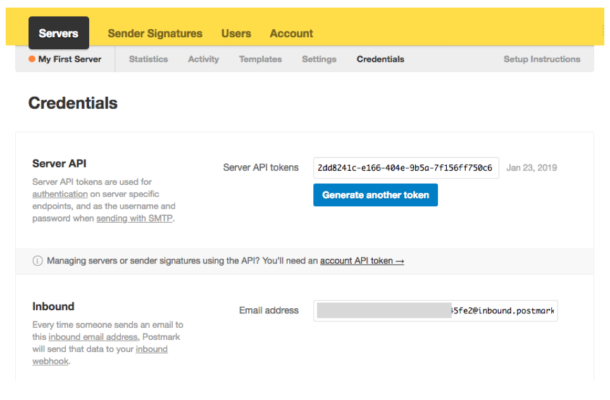
Now we are ready to go into our Laravel project and start processing inbound emails.
Set up Laravel Mailbox to receive emails #
In our Laravel project, open the AppServiceProvider
class. Here we will register how Laravel Mailbox will start receiving emails in our application. Laravel Mailbox comes with a variety of registrations that allow you to process emails from specific senders or recipients, with particular subjects and other constraints. For this tutorial, we will use the “Catch-All” registration that will enable us to process any email received.
Add the following code to the boot method in your AppServiceProvider
class:
/**
* Bootstrap any application services.
*
* @return void
*/
public function boot()
{
Mailbox::catchAll(function(InboundEmail $email) {
// Handle the incoming email
});
}
These lines are all we need to start receiving emails from Postmark using Laravel Mailbox. Isn’t that great?
To test our code, we can send an email to our Inbound Email account provided by Postmark. We can use our own email account and write a message to this recipient. In my case, I am using my personal Gmail account with the subject and body shown below.
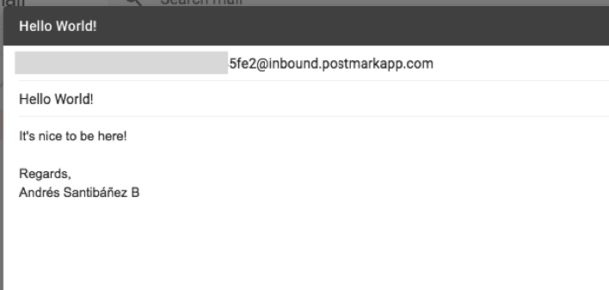
Send the email and voila! If all went well you should see an email record in the mailbox_inbound_emails
table in your project’s database. Laravel Mailbox stores automatically all incoming messages for later processing if needed. If you need to process the email when received, you can add your business logic to any Laravel Mailbox registration, in our case in the “Catch-All” registration. The InboundEmail
class contains multiple APIs that let you interact with the message subject, body, attachments and even reply to the sender.
/**
* Bootstrap any application services.
*
* @return void
*/
public function boot()
{
Mailbox::catchAll(function(InboundEmail $email) {
// Reply with a new message
// Or store and process received attachments
// Or save the message body for logging purposes
// Or anything else you'd like! :)
});
}
And that’s it! Thanks for reading.